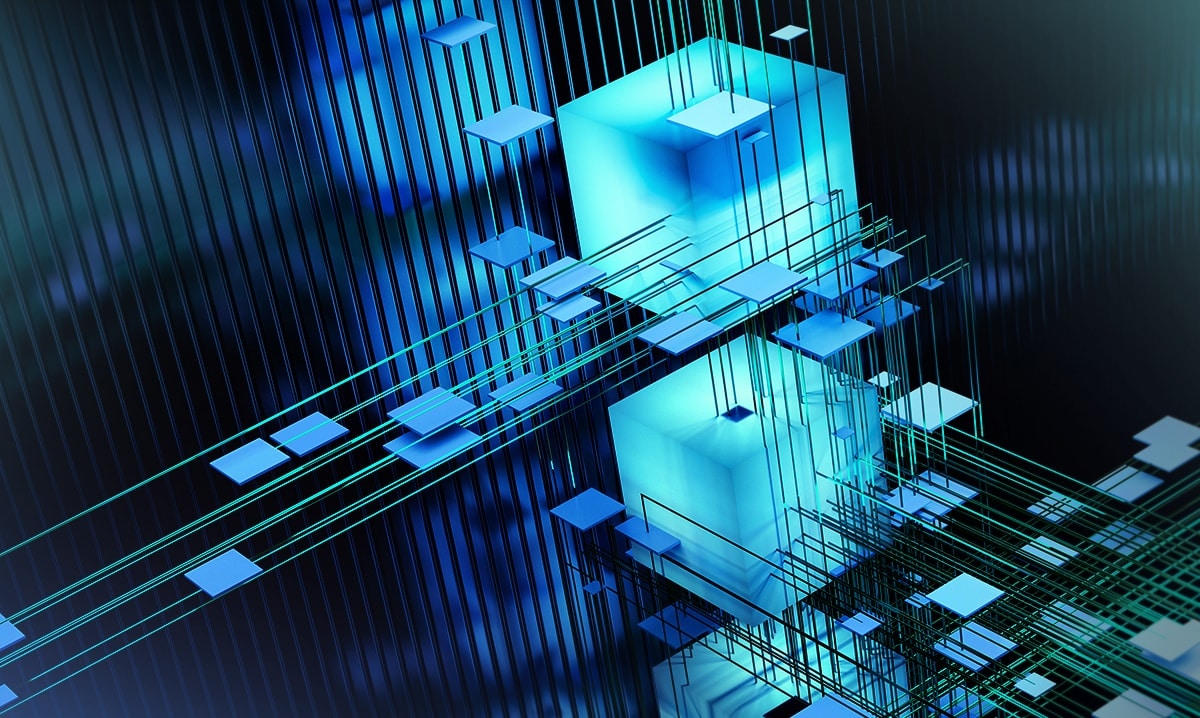
When it comes to working with arrays in Python, the ability to select and change subsets is crucial. That's where slicing comes in handy. In this article, we'll explore how to use slicing to select and change parts of an array, including columns, and take a closer look at slicing boundaries.
First, let's define what slicing is in Python. Slicing is a way to extract a portion of an array by specifying a range of indices. The syntax for slicing is as follows: array[start:end:step]. Here, start and end are integer indices that define the start and end of the slice, and step specifies the increment between each element in the slice.
To understand how slicing works, let's start with a simple example. Suppose we have an array of numbers as follows:
```
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
```
If we wanted to select only the first four elements of this array, we could use the following slice:
```
slice = numbers[0:4]
```
This would create a new array that contains the elements at indices 0, 1, 2, and 3 of the original array. We could also specify the step parameter to skip every other element in the slice:
```
slice = numbers[0:4:2]
```
This would create a new array that contains the elements at indices 0 and 2 of the original array.
Now let's apply the same principles to a two-dimensional array. Suppose we have a 2D array of numbers as follows:
```
arr = [[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]]
```
If we wanted to select only the first two rows and the first three columns of this array, we could use the following slice:
```
slice = arr[:2, :3]
```
Here, the `:` symbol indicates that we want to select all rows and columns up to the specified index. This would create a new 2D array that contains the elements in the top left corner of the original array.
We can also use slicing to assign new values to parts of an array. For example, suppose we wanted to set all the values in the first two rows and first three columns of the 2D array above to zero. We could do this with the following code:
```
arr[:2, :3] = 0
```
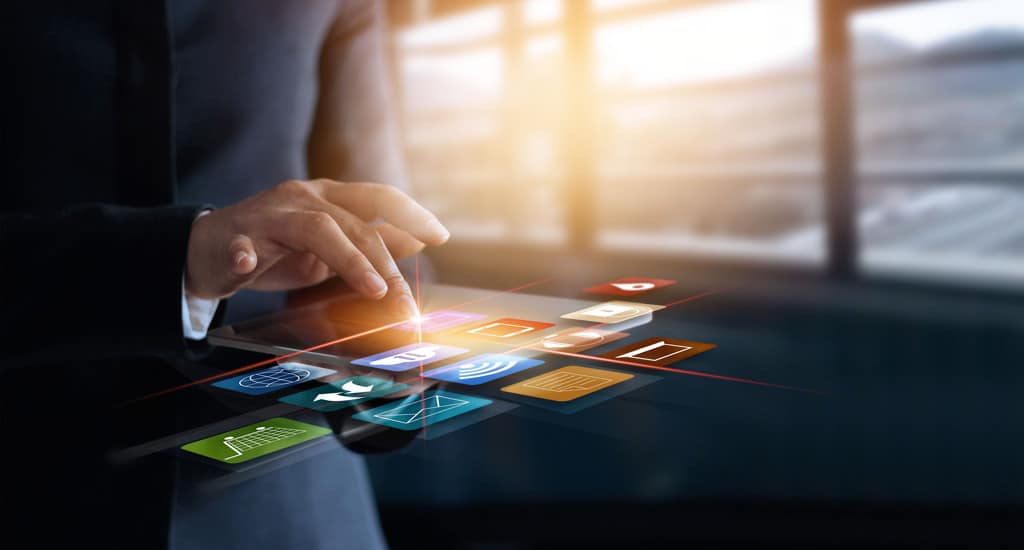
This would modify the original array so that all the elements in the top left corner are now equal to zero.
It's important to note that Python uses 0-based indexing, meaning the first element in an array has an index of 0, not 1. This can sometimes lead to confusion when working with slices, as the start and end indices may not correspond with the actual position of the elements in the array.
In conclusion, slicing is a powerful tool for working with arrays in Python. By understanding how to use slices to select and change subsets of an array, including columns and two-dimensional arrays, you can write more efficient and effective code. Just remember to be mindful of the 0-based indexing system and the boundaries of your slices.
At Mylinking, we specialize in network traffic visibility, network data visibility, and network packet visibility. Our services allow you to capture, replicate, and aggregate inline or out-of-band network traffic without packet loss. We deliver the right packets to the right tools, such as IDS, APM, NPM, monitoring, and analysis systems, to ensure a secure and efficient network environment. Contact us today to learn more about our services.